AWS EventBridge is a Serverless Event Bus service offered on the Amazon Cloud Platform that supports the implementation of various loosely coupled event driven architectures and design to fit a variety of application needs. With its simple setup and seamless API usage – publish and subscribe really – EventBridge guarantees message delivery from publishers dropping payloads onto its event buses to a range of supported targets from the all purpose Lambda function to more specialized targets like API endpoints and also including many third party vendors. Since the nature of the publishing and subscribing is relatively loosely coupled compared to some other integrations, EventBridge also supports designs where it becomes easy to swap out or replace component services in a larger system.
I have explored some of these EventBridge design paradigms in some of my other posts covering EventBridge and if you are interested please take a look at these examples on using EventBridge as the glue for an order fulfillment platform and also using it as a type specific message notification system.
Now there is one cool feature about EventBridge that may not be something that is immediately noticeable – the ability for EventBridge to be used to send cross account messages. Yes, EventBridge can be used to send messages to a completely different Amazon Account – either within your own organization or even with vendor or partner organization accounts. And the cool thing about it is from the API and SDK standpoint, there is absolutely not difference in the usage – you simply publish to or subscribe from the particular event bus the exact same way as you would for an event bus within a single account. So all EventBridge based architectures – including the examples I provided above would seamlessly work by adding or replacing one of the component services with another one from a completely separate account.
The catch – a very minimal Identity and Access Management – IAM based resource policy needs to be defined for your EventBridge bus to selectively grant permissions to the specified AWS account that will access it.
While it takes some time getting used to – it certainly did for me – IAM is great from a security standpoint – besides generally encouraging about being extremely granular in its access definitions, it also offloads much of the actual work of enforcing the security to AWS. In general you can trust Amazon to have brutally solid implementations of your IAM policies. And where you can use EventBridge cross-account, it definitely is far more seamless from an integration perspective – not access token exchanges and configuring and all that – after you setup your IAM policy, you should be good to go – which is what we are going to do next.
Defining an EventBridge Event Bus IAM Policy to grant publish access to another AWS Account
I will first demonstrate this using my preferred choice – a Cloudformation YAML – however I will also show the resultant JSON policy of the yaml definition that you can plug into the console should you wish – I will show where. But basically the points you need to note – we need to allow the type of “events:PutEvents” to the Root ARN – the amazon resource number of the root account – which we want to grant access to.
Just note the code below is referencing an event bus – I have the rest of the cloudformation template definitions for a sample application with the Lambda implementations defined in the sections below.
CrossAccountEventBusPolicy:
Type: AWS::Events::EventBusPolicy
Properties:
EventBusName: !Ref CrossAccountEventBus
StatementId: "CrossAccountEventBusStatement"
Statement:
Effect: "Allow"
Principal:
AWS: "arn:aws:iam::<ADD_ACCOUNT_ID_HERE>:root"
Action: "events:PutEvents"
Resource: !GetAtt CrossAccountEventBus.Arn
The resultant policy will be defined and viewable in the EventBridge console under the “CrossAccountEventBus” name after it is created.
It will look like this:
{
"Version": "2012-10-17",
"Statement": [{
"Sid": "CrossAccountEventBusStatement",
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<ADD_ACCOUNT_ID_HERE>:root"
},
"Action": "events:PutEvents",
"Resource": "arn:aws:events:<your_account_and_region>:event-bus/<your_event_bus_name_as_defined>"
}]
}
And can be added manually by adding to the console directly under the specific event bus by clicking manage permissions.
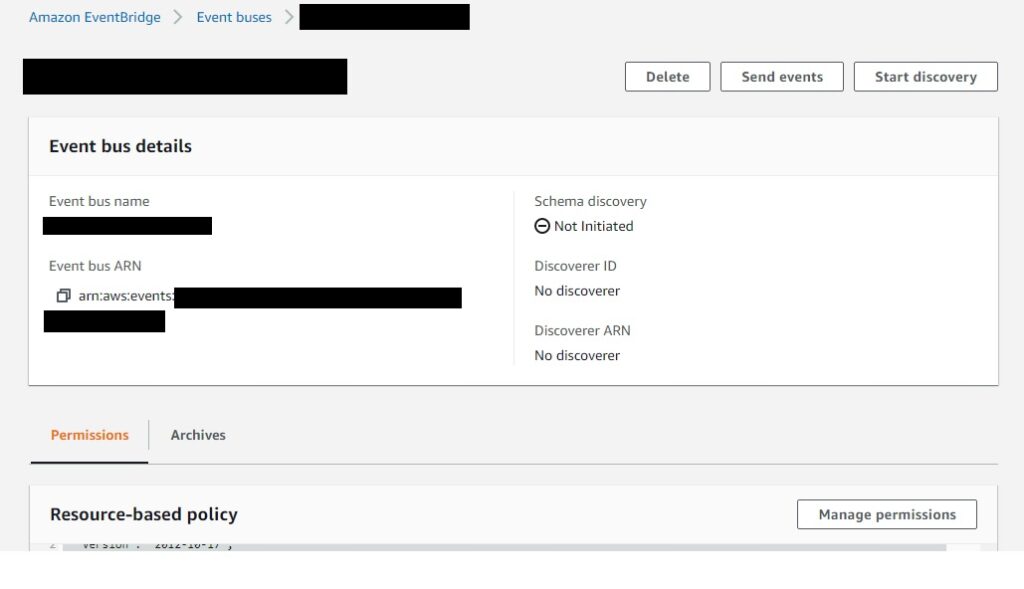
Next, lets complete the rest of the example application to demonstrate this.
Defining and coding a subscriber to the Event Bus
If you look at the image in the top of the article – we are going to complete the cloudformation yaml definition for the “AWS Account B” – which is really just an event bus, the eventbus policy which I showed above and a lambda subscribing to that event bus – this section below.
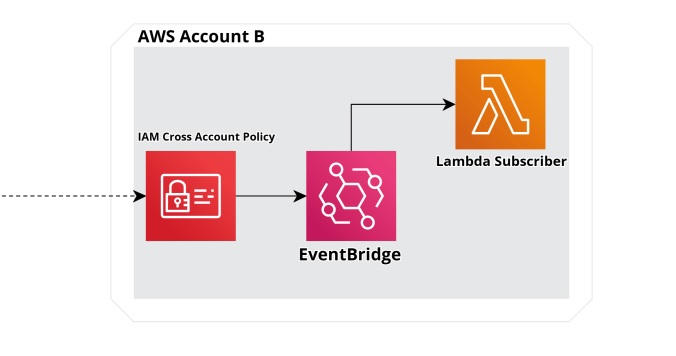
Lets tie it all together first in the yaml cloudformation template…first the yaml start and the actual event bus.
AWSTemplateFormatVersion: '2010-09-09'
Transform: AWS::Serverless-2016-10-31
Description: AWS Account B Stack - event bus, policy and subsciber lambda
Resources:
CrossAccountEventBus:
Type: AWS::Events::EventBus
Properties:
Name: "<add_a_name_for_your_bus_here>"
Next append the policy yaml from above, not doing that to avoid repetition.
And third – the subscriber Lambda which will receive events published to the bus from the “AWS Account A”.
CrossAccountMessageSubscriber:
Type: AWS::Serverless::Function
Properties:
Runtime: python3.9
CodeUri: ./cross-account-subscriber/
Handler: cross-account-subscriber.event_handler
FunctionName: cross-account-subscriber
Events:
CrossAccountMessage:
Type: EventBridgeRule
Properties:
EventBusName: !Ref CrossAccountEventBus
Pattern:
detail-type:
- cross-account-message
For demonstration purposes – this will be accepting payloads with the detail type of “cross-account-message” which we will code out publisher Lambda to push with.
For completeness – here is the simple Python code for the subscriber – we aren’t going to actually do anything with the message other than to print it.
import json
def event_handler(event, context):
cross_account_event = event["detail"]
print(f"We have got a cross account message from AWS Account A: {cross_account_event}")
Granting access to the Event Bus for the Lambda in the other account and publishing messages across accounts
This stack below will define the left half of the image on top – cropped here for convenience.
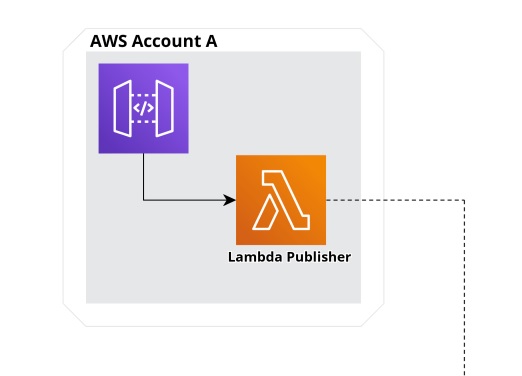
Our hypothetical stack will have a Lambda fronted by an API Gateway endpoint for accepting incoming requests which it will then publish to AWS Account B via the event bus.
This stack needs to be deployed to which ever account you specified in the IAM EventBus Policy.
AWSTemplateFormatVersion: '2010-09-09'
Transform: AWS::Serverless-2016-10-31
Description: AWS Account B Stack - event bus, policy and subsciber lambda
Resources:
CrossAccountMessagePublisher:
Type: AWS::Serverless::Function
Properties:
Runtime: python3.9
CodeUri: ./cross-account-publisher/
Handler: cross-account-publisher.lambda_handler
FunctionName: cross-account-publisher
Policies:
- AmazonEventBridgeFullAccess
Environment:
Variables:
AWS_ACCOUNT_B_EVENT_BUS_ARN: arn:aws:events:<your_account_and_region>:event-bus/<your_event_bus_name_as_defined>
Events:
HttpAPIEvent:
Type: HttpApi
Properties:
Path: /endpoint
Method: post
PayloadFormatVersion: "2.0"
Which can then publish messages in the bus as demonstrated using the Python code below.
I am using example values that would be coming from the API payload – which should be sufficient to show the gist of how to publish.
The main thing is we need to know the ARN of the event bus in the other account which we are passing to the Lambda as an environment variable.
from datetime import datetime
import boto3
import os
import json
cross_account_b_bus = os.environ["AWS_ACCOUNT_B_EVENT_BUS_ARN"]
eventbridge_client = boto3.client("events")
def lambda_handler(event, context):
event = json.loads(event["body"])
cross_account_message = {
'Time': datetime.now(),
'Source': "cross-account-publisher",
'Detail': json.dumps(event),
'DetailType': 'cross-account-message',
'EventBusName': cross_account_b_bus
}
eventbridge.put_events(
Entries=[
eventbridge_event
]
)
Closing words
To test this – modify the templates with values related to your specific accounts and deploy each respectively to their account – everything should be seamless. I use a similar setup where I collate events in a single account within an organization which has the common processing platform and there is no difference though all the messages are tagged appropriately to easily index and identify the source. Certainly it would fit in that use case such as mine where we are collating data into a single place for processing but the point I would like to reiterate again – it doesn’t matter the design – cross account publishing is a feature you can make use of as an added convenience of using EventBridge in case one of the components of the event driven system so happens to be in another account. With other solutions you would probably need to build out an API or recreate the micro service in your account and exchange credentials and so on – with EventBridge you easily define a small IAM policy which can just as easily be revoked.
Hope this was useful in helping you consider where you might need to send events between accounts in your Amazon Cloud Platform.